Several Fractal Algorithms
Kleinian Columns
Now, these shapes:
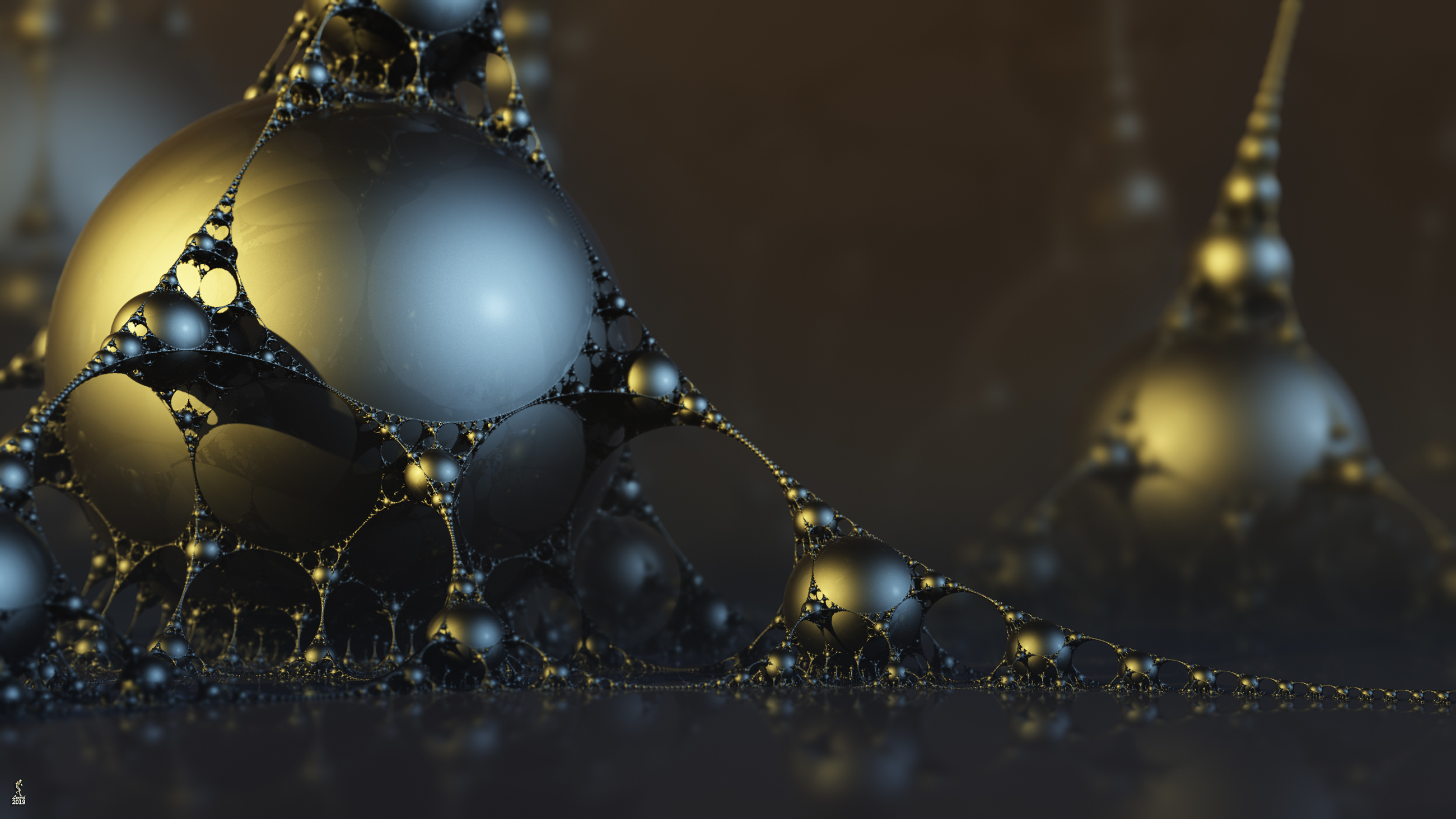
are Kleinian, and one way to draw them is using the same algorithm for drawing Apollonian gaskets, but instead of drawing circles, they're spheres. To draw these gaskets, the algorithm is draw an outer circle, and place two circles of equal size between them, so that they're tangential to all three of the circles. The second interation, in the triangular pores created by the circles fit another circle that is tangential to the other three circles. Rinse and repeat.
Glsl
Copied!
/**
c4 doesn't need to have center initialized
*/
void getZ(circle c1, circle c2, circle c3, circle c4, inout vec2 arr[4])
{
float k1 = 1.0/c1.r, k2 = 1.0/c2.r, k3 = 1.0/c3.r, k4 = 1.0/c4.r;
float x1 = c1.center.x, x2 = c2.center.x, x3 = c3.center.x;
float y1 = c1.center.y, y2 = c2.center.y, y3 = c3.center.y;
vec2 discrmnt = complexSquareRoot(k1*k2*(x1*x2-y1*y2)+k2*k3*(x2*x3-y2*y3)+k1*k3*(x1*x3-y1*y3),
k1*k2*(x1*y2+x2*y1)+k2*k3*(x2*y3+x3*y2)+k1*k3*(x1*y3+x3*y1));
arr[0] = vec2((x1*k1+x2*k2+x3*k3-2.0*discrmnt.x)/k4, (y1*k1+y2*k2+y3*k3-2.0*discrmnt.y)/k4);
arr[1] = vec2((x1*k1+x2*k2+x3*k3-2.0*discrmnt.x)/k4, (y1*k1+y2*k2+y3*k3+2.0*discrmnt.y)/k4);
arr[2] = vec2((x1*k1+x2*k2+x3*k3+2.0*discrmnt.x)/k4, (y1*k1+y2*k2+y3*k3-2.0*discrmnt.y)/k4);
arr[3] = vec2((x1*k1+x2*k2+x3*k3+2.0*discrmnt.x)/k4, (y1*k1+y2*k2+y3*k3+2.0*discrmnt.y)/k4);
}
bool testZ(circle c1, circle c2, circle c3, circle c4)
{
float k1 = 1.0/c1.r, k2 = 1.0/c2.r, k3 = 1.0/c3.r, k4 = 1.0/c4.r;
float x1 = c1.center.x, x2 = c2.center.x, x3 = c3.center.x, x4 = c4.center.x;
float y1 = c1.center.y, y2 = c2.center.y, y3 = c3.center.y, y4 = c4.center.y;
// z = x + iy
vec2 leftEquation = vec2(2.0*k1*k2*(x1*x2-y1*y2)+2.0*k1*k3*(x1*x3-y1*y3)+2.0*k1*k4*(x1*x4-y1*y4)+2.0*k2*k3*(x2*x3-y2*y3)+2.0*k2*k4*(x2*x4-y2*y4)+2.0*k3*k4*(x3*x4-y3*y4),
2.0*k1*k2*(x1*y2+x2*y1)+2.0*k1*k2*(x1*y2+x2*y1)+2.0*k1*k2*(x1*y2+x2*y1)+2.0*k1*k2*(x1*y2+x2*y1)+2.0*k1*k2*(x1*y2+x2*y1)+2.0*k1*k2*(x1*y2+x2*y1));
vec2 rightEquation = vec2(k1*k1*(x1*x1-y1*y1)+k2*k2*(x2*x2-y2*y2)+k3*k3*(x3*x3-y3*y3)+k4*k4*(x4*x4-y4*y4),
2.0*k1*k1*x1*y1+2.0*k2*k2*x2*y2+2.0*k3*k3*x3*y3+2.0*k4*k4*x4*y4);
return (leftEquation.x == rightEquation.x && leftEquation.y == rightEquation.y);
}
float getK(circle c1, circle c2, circle c3)
{
float k1 = 1.0/c1.r, k2 = 1.0/c2.r, k3 = 1.0/c3.r;
return k1+k2+k3+2.0*sqrt(k1*k2+k2*k3+k3*k1);
}
Menger Sponges
-A cube has: -6 faces -12 edges -8 vertices Menger Cube definition: Let there be an invisible unit cube at the center called U_00. Draw cubes in every unit space where that unit space is not directly adjacent to a face of U_00. Repeat this recursively as many times desired, n -l(M) = 3^n -u_1 depends on the position within the cube which depends on n -M_1 = { U of all u_1s } -M_0 = { I of all u_0s } // That is, the empty cube branch -The empty cube branch is the weird cross-y looking shape that's inside a menger cube -A Menger cube of 0 levels still has the empty root cube. -To determine if a coordinate is in 0 you could just skewer the three planes -It seems the 2mod3 or (1mod2 if starting from 0) coordinate is a whole, depending at what root you're in ∓ -------------------- Questions (in order of appearance) Q: How big is the length (in unit cubes) of the root empty cube given n? A: 3^(n-1) Q: How big in length is any subsequent empty cube node? A: The cube root of the previous level Q: What is the length of the ith empty cube node, given menger cube of n levels? // Assume i = 0 still has the empty root cube A: f(n,i) = 3^(n-i-1) Q: Given n and the currentCube's position, what is the formula for determining if currentCube is in M_0;
Pseudo
Copied!
function iterateThruSponge()
{
Let's say n = 3;
l = 3^n = 27
-We iterate the cube in i's member of I^3
-Then the parent empty cube is 9, the child is 3 the next is 1
Q: Having said that, what are the coordinates of these wholes?
A: Well, parent cube is 9x9x9 in a 27x27x27 cube, which means
the parent cube's bottom-left-front most position starts at
(9,9,9).
-Let's call the empty cube u_0_i_j. Where i is the ith level j is a member of {0,1,2,3,4}
representing which of the 5 child cubes in a node it is
-Therefore, the coordinates are:
u_0_0: (9,9,9)
u_0_1_j: (9+-2*9,9+-2*9,9+-2*9)
u_0_2_j: ((9+-2*9)+-2*3, ...)
u_0_3_j: (((9+-2*9)+-2*3)+-2*1, ...)
u_0_n_j: (3^(n-i-1), ...)
}
A: The skewer algorithm. Poke a skewer through all planes xy, xz, yz, and if a coordinate lands in there then it is in M_0. The algorithm { 1. Check if xy is a 1mod2nd coordinate, where the holes are 3^0, then where holes are 3^1, and repeat this until 3^(n-1). Repeat this for the next two planes. // Actually, lol, just do this operation for all 3 planes } Q: What would a coordinate space inside a cube look like? A: Let's say there's an (i,j,k) that are members of I^3 where (0,0,0) is the bottom-left-front most part of the cube.
-------------------- Psuedo algorithm
Pseudo
Copied!
function mengerSponge(desiredLevels)
{
for(var x,y,z = 0; ; x,y,z=goToNextSubCube())
{
drawCube.pos.set(x,y,z);
}
}
function goToNextSubCube()
{
}
function isInEmptyCubeBranch(pos)